Avatar Group
A component for grouping multiple avatars together with customizable layout and styling options.
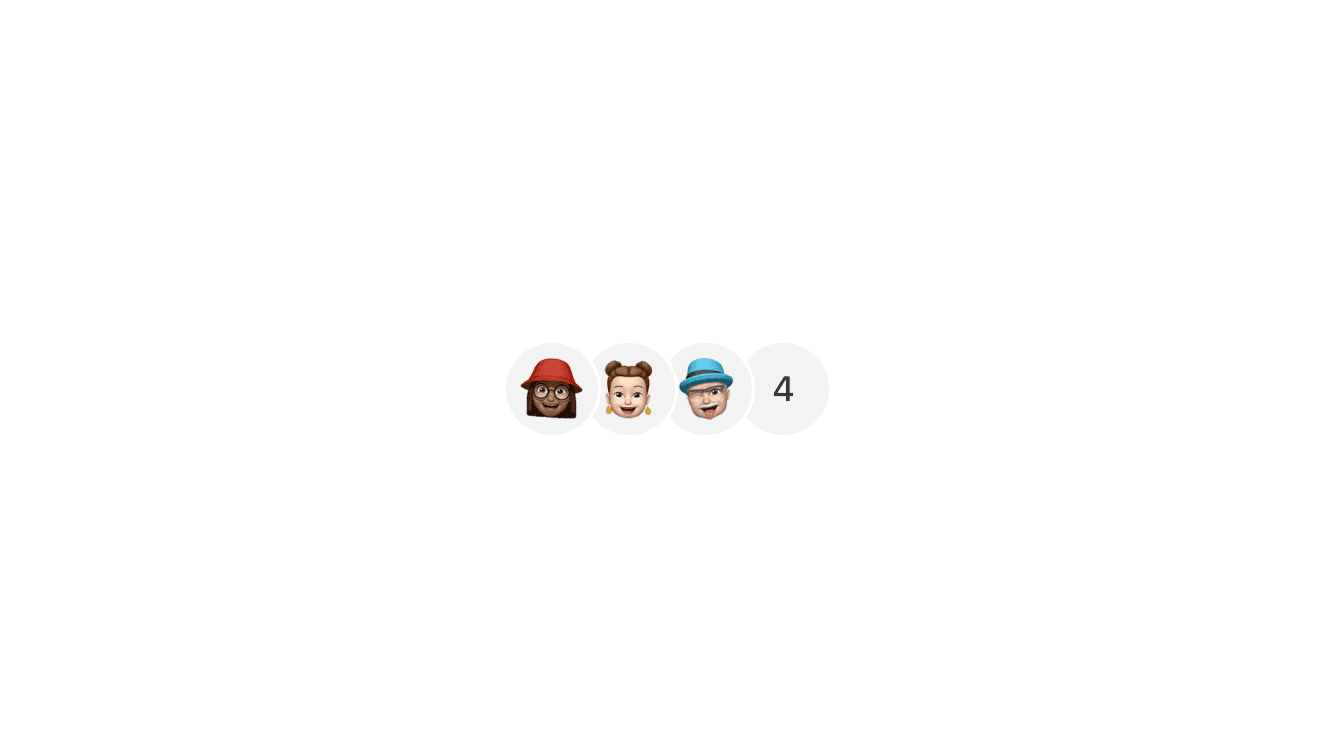
Features
- Group multiple avatars with consistent spacing
- Maximum avatar limit with overflow count
- Optional ring border around avatars
- Customizable ring color
- Consistent sizing across grouped avatars
- Square or circular shapes
Installation
npx shadcn@latest add "https://frappe-ui-react.tmls.dev/registry/native-avatar-group"
Usage
Basic Avatar Group
import { Avatar, AvatarGroup } from "@/components/ui/avatar";
export function AvatarGroupDemo() {
return (
<AvatarGroup>
<Avatar
src={{ uri: "https://example.com/avatar1.jpg" }}
name="John Doe"
/>
<Avatar
src={{ uri: "https://example.com/avatar2.jpg" }}
name="Jane Smith"
/>
<Avatar
src={{ uri: "https://example.com/avatar3.jpg" }}
name="Bob Wilson"
/>
</AvatarGroup>
);
}
With Maximum Limit
import { Avatar, AvatarGroup } from "@/components/ui/avatar";
export function AvatarGroupWithMax() {
return (
<AvatarGroup max={2}>
<Avatar name="John Doe" />
<Avatar name="Jane Smith" />
<Avatar name="Bob Wilson" />
<Avatar name="Alice Brown" />
</AvatarGroup>
);
}
Customized Ring
import { Avatar, AvatarGroup } from "@/components/ui/avatar";
export function AvatarGroupWithRing() {
return (
<AvatarGroup showRing ringColor="#e2e8f0">
<Avatar name="John Doe" />
<Avatar name="Jane Smith" />
<Avatar name="Bob Wilson" />
</AvatarGroup>
);
}
Squared Avatars
import { Avatar, AvatarGroup } from "@/components/ui/avatar";
export function SquaredAvatarGroup() {
return (
<AvatarGroup squared size="xl">
<Avatar name="John Doe" squared />
<Avatar name="Jane Smith" squared />
<Avatar name="Bob Wilson" squared />
</AvatarGroup>
);
}